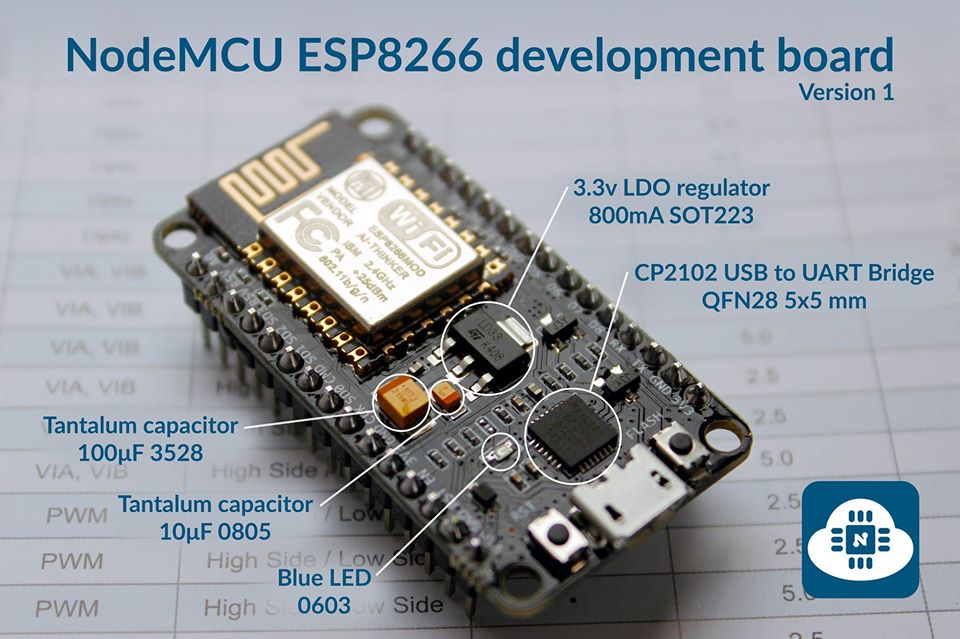
ESP8266 Introduction
2020, Jul 06
ESP8266 - Getting your MCU to blink
#define LED 2 //Define blinking LED pin
void setup() {
pinMode(LED, OUTPUT); // Initialize the LED pin as an output
}
// the loop function runs over and over again forever
void loop() {
digitalWrite(LED, HIGH); // Turn the LED on
delay(1000);
digitalWrite(LED, LOW);
delay(1000);
}
ESP8266 - Read DHT11 data
#include <DHT.h>
#include <DHT_U.h>
#define DHTTYPE DHT11
#define DHTPIN D1
DHT dht(DHTPIN, DHTTYPE);
float humidity;
float temperature;
void setup() {
Serial.begin(115200);
Serial.println("DHT11 starting ...");
dht.begin();
}
void loop() {
float converted = 0.00;
humidity = dht.readHumidity();
temperature = dht.readTemperature();
converted = (temperature * 1.8) + 32;
Serial.print("Fahrenheit = ");
Serial.print(converted);
Serial.println("F");
Serial.print("Humidity =");
Serial.println(humidity);
delay(2000);
}
Power Bus
rpi UBEC rechargeable batteries blog
Links
https://arduino-esp8266.readthedocs.io/en/latest/esp8266wifi/readme.html
https://randomnerdtutorials.com/esp8266-pinout-reference-gpios/
https://lastminuteengineers.com/esp8266-dht11-dht22-web-server-tutorial/
https://tttapa.github.io/ESP8266/Chap16%20-%20Data%20Logging.html
https://github.com/SpacehuhnTech/esp8266_deauther/tree/v2.1.0/esp8266_deauther
https://circuitdigest.com/microcontroller-projects/using-wifi-manager-on-nodemcu-to-scan-and-connect-wifi-networks
https://tttapa.github.io/ESP8266/Chap05%20-%20Network%20Protocols.html
https://github.com/esp8266/ESPWebServer
https://tttapa.github.io/ESP8266/Chap08%20-%20mDNS.html